Hidden Gems in Modern JavaScript You Should be Using: Part 2
Professional Services, Technical Blog
Last month we reviewed some hidden gems in JavaScript involving operators that help with writing more efficient, readable, and maintainable code. This month we’ll take a look at some useful functions for arrays that help in similar ways. Arrays are a very common data structure in programming, and with their use comes the need to add, find, and remove data elements.
Flattening
When working with nested arrays, there can be a need to remove the hierarchy of levels and create a flattened array with all elements at the same level. The Array.prototype.flat() function will return an array with that modification. In addition, a number can be passed to the function to flatten only to a specific number of levels:
let arr = [1, [2, [3, 4], 5], 6]; let flattened = arr.flat(); // Flattens 1 level deep console.log(flattened); // [1, 2, [3, 4], 5, 6] flattened = arr.flat(2); // Flattens 2 levels deep console.log(flattened); // [1, 2, 3, 4, 5, 6]
Many JavaScript developers are already familiar with the map function. It creates a new array populated with the results of calling a provided function on every element in the calling array. If an operation is needed to flatten in addition to map, the Array.prototype.flatMap() function combines both a map and a flat operation in a single function. In this example, the map portion of the operation returns new arrays for each of the elements in the original array, and then flattens the results into a single array:
let arr = [1, 2, 3]; let result = arr.flatMap(x => [x, x * 2]); console.log(result); // [1, 2, 2, 4, 3, 6]
Accessing Elements and Indices
For adding elements to an array at specific indices, the Array.prototype.fill() function can be used. This is handy for initializing values in an array or resetting values within a certain range of indices. The parameters passed in are the value to fill with, the starting index, and the ending index. Note that the values are updated up to the ending index, but do NOT include it:
let arr = [1, 2, 3, 4, 5]; arr.fill(0, 2, 4); // Fills indices 2 to 4 with 0 console.log(arr); // [1, 2, 0, 0, 5]
As indices are often used when working with arrays, the Array.prototype.at() function is useful for accessing an element at a specific index. This function is particularly useful as it supports negative indices, which work from the end of the array rather than the start. This makes accessing elements near the end in an array easy, as the length of the array doesn’t have to be utilized:
const arr = [10, 20, 30, 40]; console.log(arr.at(-1)); // 40 (last element)
While the filter function is often used to search through arrays for a specific element or set of elements based on a given testing function, the Array.prototype.find() function can be used if only a single element is needed. This can be more helpful than filter as it will return the first element that meets this testing criteria, rather than an array containing the element:
let arr = [5, 12, 8, 130, 44]; let found = arr.find(element => element > 10); console.log(found); // 12
Similar to find, if the index is needed rather than the value, the Array.prototype.findIndex() function returns the index of the found element rather than the element itself:
let arr = [5, 12, 8, 130, 44]; let index = arr.findIndex(element => element > 10); console.log(index); // 1
There is often a need to check whether or not an array contains a specific element. The filter function is often used here, as it returns the element if found, but the Array.prototype.includes() can also be utilized. This function simply returns a Boolean value indicating if the element was found, rather than returning the element itself (or undefined if it wasn’t found):
let arr = [1, 2, 3, 4]; console.log(arr.includes(3)); // true console.log(arr.includes(5)); // false
The Array.prototype.every() and Array.prototype.some() functions can be used to test all elements against a given expression. The every() function checks if all elements in the array pass the test, and the some() function checks if at least one element passes:
const numbers = [2, 4, 6]; const areAllEven = numbers.every(num => num % 2 === 0); // true const numbers = [1, 3, 5, 6]; const hasEven = numbers.some(num => num % 2 === 0); // true
Often there is a need to loop through all elements in an array. If both the indices and elements are needed inside a loop, the Array.prototype.entries() is useful as it returns an iterator of key-value pairs for the array elements:
const arr = ['a', 'b', 'c']; for (const [index, value] of arr.entries()) { console.log(index, value); } // Output: // 0 'a' // 1 'b' // 2 'c'
Similarly, the Array.prototype.keys() and Array.prototype.values() functions return an iterator of just the keys and just the values, respectively:
const arr = ['x', 'y', 'z']; for (const key of arr.keys()) { console.log(key); } // Output: 0, 1, 2 const arr = ['red', 'green', 'blue']; for (const value of arr.values()) { console.log(value); } // Output: 'red', 'green', 'blue'
Grouping and Ordering
The Array.prototype.groupBy() function is useful for separating elements into different collections based on a provided test function. The result is an object containing arrays based on the grouping action.
const arr = [1, 2, 3, 4, 5]; const grouped = arr.groupBy(num => (num % 2 === 0 ? 'even' : 'odd')); // { odd: [1, 3, 5], even: [2, 4] }
This function was introduced in early 2024 however, so older devices and browsers may not support it.
Sorting and reversing are common operations used in collections. For JavaScript arrays, the Array.prototype.sort() and Array.prototype.reverse() methods can be utilized; however, these modify the original array which may not be ideal. To create a new array without mutating the original, the Array.prototype.toSorted() and Array.prototype.toReversed() can be used instead:
const arr = [3, 1, 2]; const sorted = arr.toSorted(); // [1, 2, 3] console.log(arr); // [3, 1, 2] (original remains unchanged) const arr = [1, 2, 3]; const reversed = arr.toReversed(); // [3, 2, 1] console.log(arr); // [1, 2, 3] (original remains unchanged)
JavaScript Arrays are incredibly versatile, powerful, and one of the primary objects used to store a collection of data in a single variable. Beyond the commonly used methods like map, filter, and reduce, there are many newer and lesser-known functions that can help with writing cleaner and more efficient code.
From flattening arrays to accessing elements and working with indices, these functions make up a powerful set of tools for working with data. More advanced collection operations like grouping and sorting data are also easier than ever and now we can have the often-needed benefit of being able to easily preserve the integrity of the original arrays. Learning about and incorporating these hidden gems will help transform how you write and maintain code.
If you missed it, be sure to check out Hidden Gems in Modern JavaScript You Should be Using: Part 1
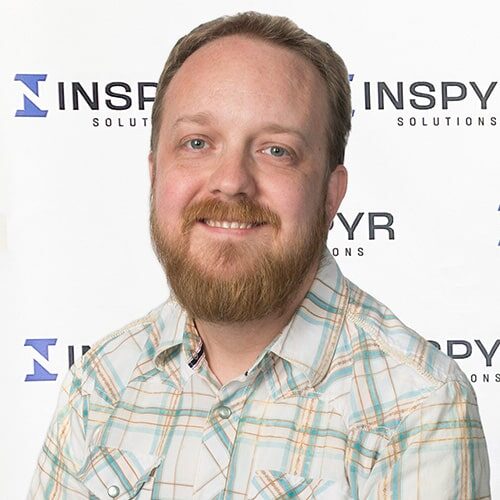
Jeremy Walker
Jeremy Walker is a senior software engineer with over 13 years of experience. He specializes in building websites and mobile apps using JavaScript. A tech enthusiast at heart, he also enjoys all things technology, gaming, and exploring the outdoors in his free time. For inquiries, you can reach him at jwalker@inspyrsolutions.com.
Share This Article
Contact Us
We’re here for you when you need us. How can we help you today?